Understanding Lists, Tuples, Sets, and Dictionaries in Python: A Beginner-Friendly Guide
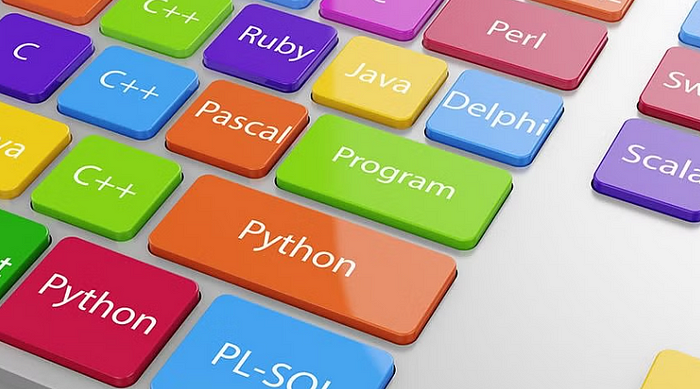
As a beginner in Python (or any programming language), you may often get confused when seeing different types of brackets — ()
, []
, and {}
—being used. You might wonder:
- When should I use square brackets
[]
? - Why do dictionaries use
{}
but access elements with[]
? - Are tuples and lists the same?
This blog post will break everything down into simple concepts, so you’ll never be confused again!
🛠️ Understanding Different Brackets in Python
1️⃣ Lists ([]
) – Ordered, Mutable Collection
A list is an ordered collection that allows duplicates and can be modified.
✅ How to Create a List?
my_list = [1, 2, 3]
✅ How to Modify a List?
my_list.append(4) # Adds 4 to the list
💡 Why ()
in .append()
?
.append()
is a method, and methods in Python always use()
.
✅ When to Use Lists?
- When you need an ordered sequence of items.
- When you need a mutable collection (i.e., you can add, remove, or change elements).
2️⃣ Tuples (()
) – Ordered, Immutable Collection
A tuple is just like a list, but it cannot be changed (immutable).
✅ How to Create a Tuple?
my_tuple = (1, 2, 3)
❌ Trying to Modify a Tuple Will Fail!
my_tuple[0] = 100 # ❌ Error: 'tuple' object does not support item assignment
✅ When to Use Tuples?
- When you need a fixed sequence of values that should not be modified.
- Tuples are often used to represent constant data.
3️⃣ Sets ({}
) – Unordered, Unique Collection
A set is an unordered collection that does not allow duplicate values.
✅ How to Create a Set?
my_set = {1, 2, 3}
✅ How to Add Elements to a Set?
my_set.add(4) # Adds 4 to the set
💡 Why ()
in .add()
?
- Just like
.append()
,.add()
is a method, and methods always use()
.
✅ When to Use Sets?
- When you need unique values only.
- When you don’t care about the order.
4️⃣ Dictionaries ({}
) – Key-Value Pair Collection
A dictionary is a collection of key-value pairs.
✅ How to Create a Dictionary?
my_dict = {"a": 1, "b": 2}
✅ How to Add or Modify Elements?
my_dict["c"] = 3 # Adds key "c" with value 3
💡 Why []
Instead of ()
?
- Methods like
.get()
use()
because they perform an action. - Dictionary keys are accessed with
[]
, just like lists.
✅ When to Use Dictionaries?
- When you need fast lookups based on keys.
- When you need to store key-value pairs.
🔥 Python Data Types Cheat Sheet
Lists ([]
)
- Ordered ✅
- Mutable ✅ (can add, remove, modify elements)
- Allows Duplicates ✅
- Example:
my_list = [1, 2, 3]
Tuples (()
)
- Ordered ✅
- Immutable ❌ (cannot change values after creation)
- Allows Duplicates ✅
- Example:
my_tuple = (1, 2, 3)
Sets ({}
)
- Unordered ❌
- Mutable ✅ (can add/remove elements, but not modify existing ones)
- Allows Duplicates ❌
- Example:
my_set = {1, 2, 3}
Dictionaries ({}
)
- Ordered (Python 3.7+) ✅
- Mutable ✅
- Keys Must Be Unique ❌
- Example:
my_dict = {"a": 1, "b": 2}
Here’s The Ultimate Comparison Table in a Medium-friendly format (using bullet points instead of a table):
🔥 The Ultimate Comparison of Lists, Tuples, Sets, and Dictionaries
✅ Lists ([]
)
- Ordered: ✅ Yes
- Mutable: ✅ Yes (can modify elements)
- Allows Duplicates: ✅ Yes
- Access Elements:
list[index]
- Use Case: When you need a changeable sequence of elements.
- Example:
my_list = [1, 2, 3]
my_list.append(4) # Adds 4 to the list
✅ Tuples (()
)
- Ordered: ✅ Yes
- Mutable: ❌ No (cannot modify after creation)
- Allows Duplicates: ✅ Yes
- Access Elements:
tuple[index]
- Use Case: When you need an unchangeable sequence of elements.
- Example:
my_tuple = (1, 2, 3)
# my_tuple[0] = 100 # ❌ Error: Tuples are immutable
✅ Sets ({}
)
- Ordered: ❌ No (unordered collection)
- Mutable: ✅ Yes (can add/remove elements but not modify existing ones)
- Allows Duplicates: ❌ No (only unique values)
- Access Elements: ❌ Not possible (because it’s unordered)
- Use Case: When you need a collection of unique values.
- Example:
my_set = {1, 2, 3}
my_set.add(4) # Adds 4 to the set
✅ Dictionaries ({}
)
- Ordered (Python 3.7+): ✅ Yes (keys are in insertion order)
- Mutable: ✅ Yes (can modify key-value pairs)
- Keys Must Be Unique: ✅ Yes
- Access Elements:
dict["key"]
- Use Case: When you need key-value storage with fast lookups.
- Example:
my_dict = {"a": 1, "b": 2}
my_dict["c"] = 3 # Adds key "c" with value 3
🏆 Final Takeaways
- Use
[]
for lists (mutable, ordered collections). - Use
()
for tuples (immutable, ordered collections). - Use
{}
for sets (unordered, unique values). - Use
{}
for dictionaries (key-value pairs, but access elements with[]
). - Methods always use
()
, whether it's.append()
,.add()
, or.get()
.
By understanding these concepts, you’ll be more confident in handling Python collections. Happy coding! 🚀
💡 Found this helpful? Share this post with your fellow Python learners! 😊